- Create a new WPF Project.
- Rename the Main window to DataGridSample.xaml( Make sure you rename the StartupUri in App.xaml too).
- Drag and drop DataGrid to this window and Name it ProductDataGrid.
- Create a table named "Products" in your Database( I use SQL Server 2008)
- Create Model Classes with the Entity Framework. Create Model.
- Create an instance of this Model in your Codebehind class.(Here the ProductEntity)
- Use this LINQ query to get the ProductName and ProductID
- We have to bind the DataGrid columns are bind to ProdID and ProdName, so we have to add this code to the DataGrid Control in the DataGridSample.xaml
- Use DataContext to bind DataGrid to "prod".
<DataGrid Name="ProductDataGrid" AutoGenerateColumns="False" Height="120" Width="350" Margin="10" />
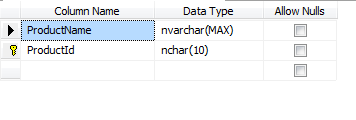
ProductEntities _Product = new ProductEntities();
var prod = from p in _customer.Products
select new { ProdId = p.ProductId, ProdName = p.ProductName };
<DataGrid Name="ProductDataGrid" AutoGenerateColumns="False" Height="120" Width="350" Margin="10" ItemsSource="{Binding}" >
<DataGrid.Columns>
<DataGridTextColumn Header=" Product Name" Binding="{Binding ProdId}"/>
<DataGridTextColumn Header=" Product Name" Binding="{Binding ProdName}"/>
</DataGrid.Columns>
</DataGrid>
ProductDataGrid.DataContext = prod;
DataBindingSample.cs
------------------------
public partial class DataBindingSample: Window
{
//Model class Instance.
ProductEntities _Product;
public DataBindingSample()
{
InitializeComponent();
_Product = new ProductEntities();
var prod = from p in _Product.Products
select new { ProdId = p.ProductId,
ProdName = p.ProductName };
ProductDataGrid.DataContext = prod;
}
}
DataBindingSample.xaml
------------------------
<StackPanel>
<DataGrid Name="ProductDataGrid" AutoGenerateColumns="False" Height="120" Width="300" Margin="10" ItemsSource="{Binding}" >
<DataGrid.Columns>
<DataGridTextColumn Header=" Product ID" Binding="{Binding ProdId}"/>
<DataGridTextColumn Header=" Product Name" Binding="{Binding ProdName}"/>
</DataGrid.Columns>
</DataGrid>
</StackPanel>
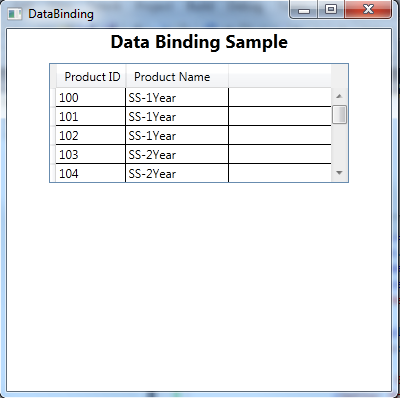
0 comments:
Post a Comment